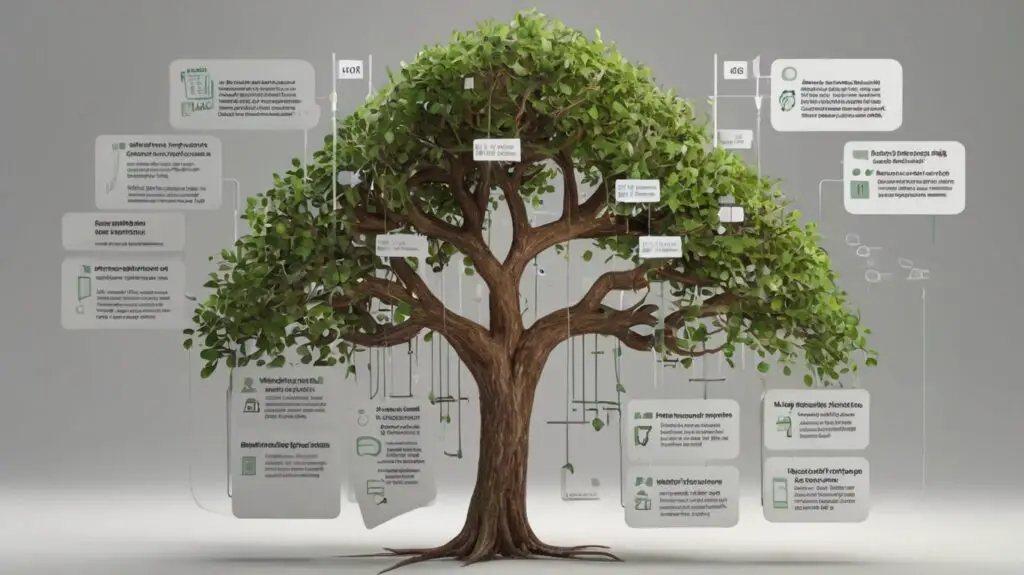
In the ever-evolving landscape of artificial intelligence and data science, decision tree machine learning algorithms have emerged as powerful tools for solving complex problems and making data-driven decisions. These versatile algorithms have found applications across various industries, from finance to healthcare, and continue to revolutionize the way we analyze and interpret data. In this comprehensive guide, we’ll dive deep into the world of decision trees, exploring their inner workings, advantages, limitations, and real-world applications.
Introduction: The Root of Decision Trees
At their core, decision trees are a type of supervised machine learning algorithm used for both classification and regression tasks. Imagine a flowchart-like structure where each internal node represents a “test” on an attribute, each branch represents the outcome of the test, and each leaf node represents a class label or a numerical value. This tree-like model of decisions and their possible consequences makes it an intuitive and powerful tool for decision-making processes.
The importance of decision trees in machine learning cannot be overstated. They provide a transparent and interpretable way to make predictions, which is crucial in fields where understanding the decision-making process is as important as the outcome itself. From predicting customer behavior to diagnosing diseases, decision trees have found their place in various domains, including finance, healthcare, marketing, and more.
How Decision Trees Work: Branching Out into Understanding
To truly appreciate the power of decision trees, it’s essential to understand their basic structure and how they make decisions. Let’s break it down:
The Anatomy of a Decision Tree
- Root Node: This is the topmost node in the tree, representing the entire dataset.
- Internal Nodes: These are the decision nodes that test an attribute and split the data.
- Branches: These connect nodes, representing the outcome of a test.
- Leaf Nodes: These are the terminal nodes that represent the final output (class or value).
The Decision-Making Process
Imagine you’re trying to predict whether a customer will churn or not. A simple decision tree might look like this:
- Root Node: “Has the customer been with us for more than 2 years?”
- If Yes, go to Branch A
- If No, go to Branch B
- Branch A: “Does the customer use more than 3 of our products?”
- If Yes, Leaf Node: Low churn risk
- If No, go to Branch C
- Branch B: “Has the customer contacted support in the last month?”
- If Yes, Leaf Node: High churn risk
- If No, Leaf Node: Medium churn risk
- Branch C: “Is the customer’s monthly spend above $100?”
- If Yes, Leaf Node: Medium churn risk
- If No, Leaf Node: High churn risk
This simple example illustrates how a decision tree makes predictions by asking a series of questions and following the appropriate branches based on the answers.
The Science Behind the Splits
The key to building an effective decision tree lies in choosing the right attributes to split on at each node. This is where concepts like information gain, entropy, and Gini impurity come into play.
- Entropy measures the impurity or uncertainty in the data set.
- Information Gain is the decrease in entropy after a dataset is split on an attribute.
- Gini Impurity is another measure of how often a randomly chosen element would be incorrectly labeled if it were randomly labeled according to the distribution of labels in the subset.
The goal is to maximize information gain (or minimize Gini impurity) at each split, ensuring that the tree becomes more and more certain about its predictions as it grows.
Types of Decision Trees: A Forest of Possibilities
Decision trees come in different flavors, each suited for specific types of problems:
Classification Trees
These are used when the target variable is categorical. For example, predicting whether an email is spam or not, or classifying an image as a cat or dog.
Regression Trees
When the target variable is continuous, regression trees are used. They can predict values like house prices, stock prices, or temperature.
Ensemble Methods
While not strictly decision trees, ensemble methods like Random Forests and Gradient Boosting use multiple decision trees to improve prediction accuracy and robustness. These methods combine the predictions of several trees to produce more accurate results.
Advantages of Decision Trees: Branching Out with Benefits
Decision trees offer several advantages that make them a popular choice among data scientists and machine learning practitioners:
- Easy to Understand and Interpret: The tree structure mimics human decision-making, making it intuitive even for non-technical stakeholders.
- Handles Both Numerical and Categorical Data: Unlike some algorithms that require numerical data, decision trees can work with both types seamlessly.
- Requires Minimal Data Preparation: They can handle missing values and don’t require normalization or scaling of features.
- Efficient in Handling Large Datasets: Decision trees can process large amounts of data relatively quickly.
- Implicit Feature Selection: The tree-building process automatically identifies the most important features for prediction.
- Non-Parametric: Decision trees make no assumptions about the underlying distribution of the data.
Limitations of Decision Trees: Pruning the Drawbacks
While decision trees are powerful, they’re not without their limitations:
- Overfitting: Decision trees can create overly complex trees that don’t generalize well to new data. This is often addressed through pruning or setting constraints on tree growth.
- Biased Results for Imbalanced Datasets: If some classes dominate, the tree might be biased towards the majority classes.
- Instability: Small variations in the data can result in a completely different tree being generated. This instability is often addressed by ensemble methods.
- Greedy Approach: The algorithm makes the locally optimal choice at each node, which doesn’t always lead to the global optimum.
- Difficulty with XOR Problems: Decision trees can struggle with problems that require complex combinations of features.
Real-world Applications: From Roots to Fruits
Decision trees have found applications across various industries, demonstrating their versatility and power:

Finance
- Credit Scoring: Banks use decision trees to assess the creditworthiness of loan applicants.
- Fraud Detection: Identifying potentially fraudulent transactions based on various attributes.
Healthcare
- Disease Diagnosis: Decision trees can help in diagnosing diseases based on symptoms and test results.
- Risk Assessment: Predicting the likelihood of certain health conditions based on patient data.
Marketing
- Customer Segmentation: Grouping customers based on their characteristics and behaviors.
- Churn Prediction: Identifying customers who are likely to leave a service.
Environmental Science
- Species Identification: Classifying plant or animal species based on their characteristics.
- Climate Prediction: Forecasting weather patterns or climate change impacts.
Manufacturing
- Quality Control: Identifying factors that contribute to product defects.
- Predictive Maintenance: Determining when machinery is likely to require maintenance.
Building a Decision Tree: Growing Your Own Model
Creating a decision tree involves several steps:
- Data Preparation: Gather and clean your dataset, handling missing values and encoding categorical variables if necessary.
- Choosing the Algorithm: Select an appropriate algorithm (e.g., ID3, C4.5, CART) based on your problem and data type.
- Setting Hyperparameters: Define parameters like maximum depth, minimum samples per leaf, and minimum samples for a split.
- Training the Model: Use your prepared data to build the tree.
- Pruning: Optionally, prune the tree to prevent overfitting.
- Evaluation: Test the model’s performance on a separate validation set.
Popular libraries like scikit-learn in Python make it easy to implement decision trees. Here’s a simple example:
from sklearn.tree import DecisionTreeClassifier
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
# Load data
iris = load_iris()
X, y = iris.data, iris.target
# Split data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Create and train the model
clf = DecisionTreeClassifier(max_depth=3)
clf.fit(X_train, y_train)
# Make predictions
predictions = clf.predict(X_test)
# Evaluate the model
accuracy = clf.score(X_test, y_test)
print(f"Model Accuracy: {accuracy}")
This simple code snippet demonstrates how easy it is to get started with decision trees using scikit-learn.
Conclusion: The Evergreen Nature of Decision Trees
As we’ve explored, decision tree machine learning algorithms offer a powerful, interpretable, and versatile approach to solving complex problems across various domains. Their intuitive nature, coupled with their ability to handle different types of data, makes them an indispensable tool in any data scientist’s toolkit.
While they have limitations, ongoing research and ensemble methods continue to address these challenges, ensuring that decision trees remain relevant in the ever-evolving landscape of machine learning. Whether you’re a seasoned data scientist or just starting your journey in machine learning, understanding and leveraging decision trees can significantly enhance your ability to derive meaningful insights from data and make informed decisions.
As you continue your exploration of machine learning algorithms, remember that decision trees are just one branch of a vast and growing field. The key to mastery lies in understanding the strengths and weaknesses of each approach and knowing when and how to apply them effectively.
Thanks for reading!
If you enjoyed this article and would like to receive notifications for my future posts, consider subscribing . By subscribing, you’ll stay updated on the latest insights, tutorials, and tips in the world of data science.
Additionally, I would love to hear your thoughts and suggestions. Please leave a comment with your feedback or any topics you’d like me to cover in upcoming blogs. Your engagement means a lot to me, and I look forward to sharing more valuable content with you.
Subscribe and Follow for More