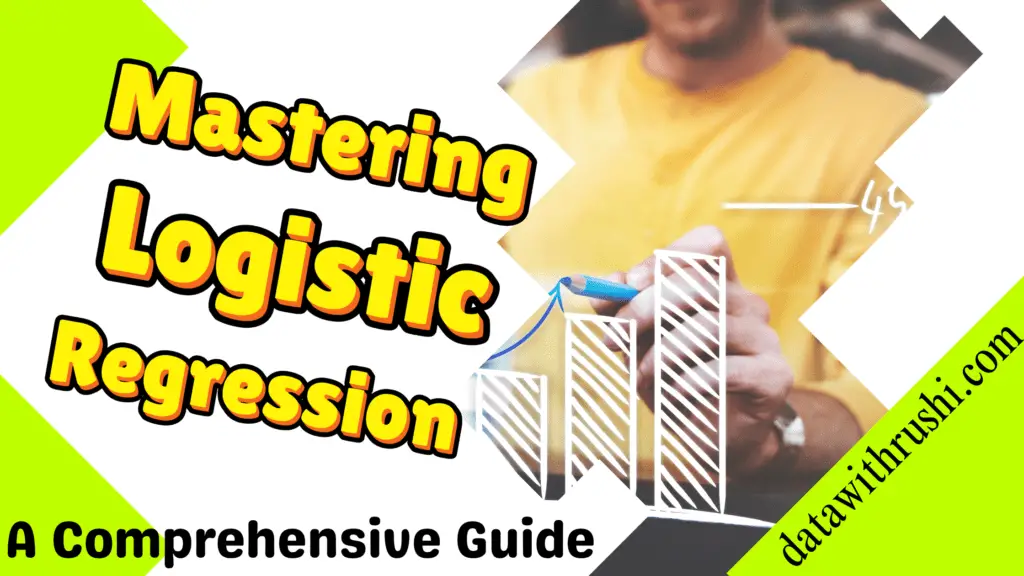
Understanding Logistic Regression: A Comprehensive Guide for Data Enthusiasts
Introduction
In the realm of data science, the ability to make accurate predictions is paramount. Whether it’s predicting customer behavior, diagnosing diseases, or assessing financial risks, the tools and techniques used must be both robust and reliable. One such technique that stands out is Logistic Regression. Unlike linear regression, which is used for continuous outcomes, logistic regression is the go-to method for predicting categorical outcomes, typically in the form of binary classifications. In this guide, we will delve deep into the world of logistic regression, exploring its core concepts, applications, implementation in Python, and more.
What is Logistic Regression?
At its core, Logistic regression is fundamentally a statistical technique that models the likelihood of a binary result depending on one or more predictor variables. This method is particularly useful when the dependent variable is categorical in nature, such as “yes” or “no”, “success” or “failure”, and “spam” or “not spam”.
The Logistic Function
The key to understanding logistic regression lies in the logistic function (also known as the sigmoid function). Unlike linear regression, which predicts a continuous outcome, logistic regression outputs probabilities constrained between 0 and 1. The logistic function is expressed as:
The logistic function is expressed as:
Here, P(Y=1|X) represents the probability of the event occurring (e.g., the probability of a customer making a purchase), and the parameters β0, β1, …, βn are estimated from the data.
Comparison with Linear Regression
While linear regression models the relationship between a dependent variable and one or more independent variables by fitting a linear equation, logistic regression models the relationship using the logistic function. The main difference lies in the output: linear regression predicts continuous outcomes, while logistic regression predicts probabilities, making it ideal for classification problems.
Applications of Logistic Regression
Logistic regression has a wide range of applications across various industries. Its versatility and ease of interpretation make it a popular choice for many data-driven decision-making processes.
1. Healthcare
In the healthcare industry, logistic regression is commonly used for disease diagnosis. For instance, predicting whether a patient has a particular disease based on various factors such as age, sex, blood pressure, and cholesterol levels. By analyzing historical patient data, logistic regression can help in early diagnosis, potentially saving lives.
2. Finance
In finance, logistic regression is employed to assess credit risk. Financial institutions use this technique to predict the likelihood of a borrower defaulting on a loan based on their credit history, income, and other relevant factors. This helps in making informed lending decisions and managing financial risks effectively.
3. Marketing
Marketers use logistic regression to predict customer behavior. For example, it can help in determining the likelihood of a customer responding to a marketing campaign or purchasing a product. By analyzing customer data, companies can tailor their strategies to target the right audience, thereby increasing conversion rates.
Case Study: Predicting Customer Churn
Consider a telecommunications company that wants to predict customer churn (i.e., whether a customer will leave the service). By applying logistic regression, the company can analyze factors such as customer satisfaction, service usage, and billing history to predict the probability of churn. This allows the company to take proactive measures to retain customers.
How to Implement Logistic Regression in Python
Implementing logistic regression in Python is straightforward, thanks to libraries like scikit-learn. Below is a step-by-step guide to getting you started.
Step 1: Import Necessary Libraries
import numpy as np
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
from sklearn.metrics import accuracy_score, confusion_matrix, classification_report
Step 2: Load the Dataset
data = pd.read_csv('your_dataset.csv')
X = data[['feature1', 'feature2', 'feature3']] # Replace with your features
y = data['target'] # Replace with your target variable
Step 3: Split the Data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
Step 4: Train the Model
model = LogisticRegression()
model.fit(X_train, y_train)
Step 5: Make Predictions
y_pred = model.predict(X_test)
Step 6: Evaluate the Model
print("Accuracy:", accuracy_score(y_test, y_pred))
print("Confusion Matrix:\n", confusion_matrix(y_test, y_pred))
print("Classification Report:\n", classification_report(y_test, y_pred))
Explanation of Each Step
- Importing Libraries: We import essential libraries such as NumPy for numerical operations, pandas for data manipulation, and scikit-learn for machine learning algorithms.
- Loading the Dataset: The dataset is loaded into a pandas DataFrame, and features (independent variables) and target (dependent variable) are defined.
- Splitting the Data: The data is split into training and testing sets to evaluate the model’s performance on unseen data.
- Training the Model: We use the LogisticRegression class from scikit-learn to train the model on the training data.
- Making Predictions: The model is used to predict the target variable on the test data.
- Evaluating the Model: Finally, we evaluate the model’s performance using accuracy, confusion matrix, and classification report.
Benefits of Logistic Regression
Logistic regression is favored for several reasons, making it a staple in the data science toolkit.
1. Ease of Interpretation
One of the primary advantages of logistic regression is its interpretability. The coefficients (β values) in the logistic regression model represent the log odds of the outcome. This makes it easy to understand how changes in predictor variables affect the probability of the outcome.
2. Efficiency with Small Datasets
Even with relatively small datasets, logistic regression exhibits strong performance. Unlike more complex models that require vast amounts of data, logistic regression can yield reliable results with limited data, making it a practical choice for many real-world applications.
3. Flexibility
Despite its simplicity, logistic regression is quite flexible. It can handle multiple predictors, including continuous, categorical, and interaction terms. This versatility allows it to be applied to a wide range of problems.
Limitations of Logistic Regression
While logistic regression is a powerful tool, it does have its limitations.
1. Assumption of Linearity
Logistic regression assumes a linear relationship between the predictor variables and the log odds of the outcome. The model might not function well if this assumption is broken. In such cases, more advanced techniques like decision trees or neural networks might be more appropriate.
2. Sensitivity to Outliers
Outliers can have a significant impact on the logistic regression model, potentially skewing the results. It’s important to preprocess the data carefully, removing or appropriately handling outliers to ensure the model’s robustness.
3. Limited to Binary Outcomes
Traditional logistic regression is designed for binary outcomes. While there are extensions like multinomial logistic regression for multiclass problems, they can be more complex to implement and interpret.
Conclusion
Logistic regression remains a fundamental tool in the arsenal of data scientists and machine learning practitioners. Its simplicity, ease of interpretation, and efficiency make it a go-to method for many binary classification problems. Whether you’re diagnosing diseases, predicting customer behavior, or assessing financial risks, logistic regression offers a reliable and interpretable solution.
We encourage you to experiment with logistic regression on your own datasets, exploring its full potential. Don’t hesitate to dive deeper into more complex models if your problem requires it, but always keep logistic regression in mind as a strong starting point.
FAQ
Q1: What is logistic regression?
A: Logistic regression is a statistical method used for binary classification that predicts the probability of an outcome based on one or more predictor variables.
Q2: How does logistic regression differ from linear regression?
A: While linear regression predicts continuous outcomes, logistic regression predicts categorical outcomes, typically a binary result like 0 or 1.
Q3: What are some common applications of logistic regression?
A: Logistic regression is widely used in fields like healthcare (e.g., predicting disease presence), finance (e.g., credit scoring), and marketing (e.g., customer churn prediction).
Q4: Can I implement logistic regression in Python?
A: Yes, you can easily implement logistic regression in Python using libraries like scikit-learn. Our blog post includes a step-by-step guide to help you get started.
Q5: What are the advantages of using logistic regression?
A: Logistic regression is easy to implement, efficient to train, and provides interpretable results. It’s particularly useful for binary classification tasks.
Q6: Are there any limitations to logistic regression?
A: Yes, logistic regression assumes a linear relationship between the independent variables and the log-odds of the dependent variable. It can also struggle with multicollinearity and non-linear problems.
Q7: Can logistic regression handle multi-class classification problems?
A: While logistic regression is primarily used for binary classification, extensions like multinomial logistic regression can handle multi-class classification problems.
Q8: Where can I find more resources to learn about logistic regression?
A: Our blog post includes a comprehensive guide and additional resources. You can also explore online courses, textbooks, and tutorials on data science and machine learning.
Q9: How can I address the limitations of logistic regression?
A: Techniques like feature engineering, regularization, and exploring alternative models (e.g., decision trees, neural networks) can help address some limitations.
Q10: Do you offer any hands-on examples or datasets for practice?
A: Yes, our blog post includes code snippets and examples. We encourage you to try implementing logistic regression with your own dataset and share your results with us!
Thanks for reading!
If you enjoyed this article and would like to receive notifications for my future posts, consider subscribing . By subscribing, you’ll stay updated on the latest insights, tutorials, and tips in the world of data science.
Additionally, I would love to hear your thoughts and suggestions. Please leave a comment with your feedback or any topics you’d like me to cover in upcoming blogs. Your engagement means a lot to me, and I look forward to sharing more valuable content with you.
Subscribe and Follow for More